Digital books in EPUB format are widely used in the world of e-books because of their compatibility, flexibility, and ease of use. However, there are times when you might want to update the metadata of an EPUB file, such as the author’s name, title, description, or even adding a custom tag. Python, being a versatile programming language, makes this process straightforward. If you’re interested in learning how to edit the metadata of EPUB files using Python, this article will guide you through the process in an engaging and efficient way.
What is EPUB Metadata?
Metadata in an EPUB file is structured information that provides details about the book, such as:
- Title: The name of the book.
- Author: The name(s) of the author(s).
- Description: A brief summary of the content.
- Publisher: The organization or person responsible for publishing the book.
- Language: The language in which the book is written.
- Custom tags: Additional information tailored to specific needs.
By editing the metadata, you can refine how the book is displayed in e-readers or categorized in a library.
Python Tools for Editing EPUB Metadata
To edit EPUB metadata using Python, you can use libraries such as EbookLib, a popular Python library designed for creating, reading, and modifying EPUB files. This library simplifies the process of working with EPUB files, offering easy access to their metadata.
Prerequisites
Before we dive into the code, ensure the following:
- Python is installed on your system.
- The EbookLib library is installed. You can install it using the command:
pip install EbookLib
. - You have an EPUB file on hand for testing purposes.
Editing Metadata Using Python
Let’s walk through the steps needed to edit an EPUB file’s metadata:
1. Import Necessary Modules
First, import the required modules from the EbookLib library in your Python script:
from ebooklib import epub
2. Open the EPUB File
Load the EPUB file that you want to modify. For example:
book = epub.read_epub('sample.epub')
3. Access and Edit Metadata
The metadata of an EPUB file is stored in its metadata attribute. Here’s how you can access it and modify fields such as the title and author:
# Access existing metadata
current_title = book.get_metadata('DC', 'title')
current_author = book.get_metadata('DC', 'creator')
# Update metadata
book.set_metadata('DC', 'title', 'New Book Title')
book.set_metadata('DC', 'creator', 'John Doe')
In this example, the title is updated to New Book Title and the author is set as John Doe.
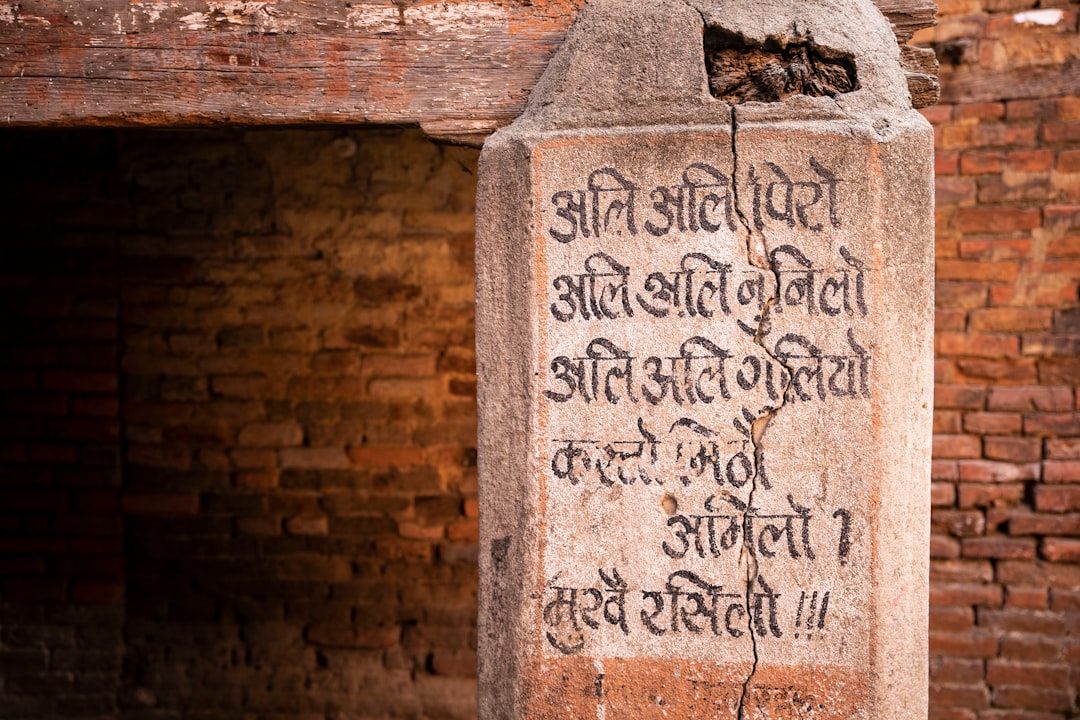
4. Save the Updated EPUB
Once you’re done editing the metadata, save the updated EPUB file. Here’s how:
epub.write_epub('updated_sample.epub', book)
This writes the updated book metadata to a new file called updated_sample.epub.
Additional Tweaks
Beyond editing the title and author, you can modify other metadata fields such as:
- Description: Provide an engaging summary of the book using
book.set_metadata('DC', 'description', 'This is an amazing book.')
. - Publisher: Update publishing information as needed with
book.set_metadata('DC', 'publisher', 'New Publisher')
. - Custom Tags: Add custom tags by specifying a new field name and value in the same format.
Why Use Python for Metadata Editing?
Python is ideal for metadata management due to its flexibility and wide range of libraries. For tasks involving batch processing or automation of metadata modification across multiple EPUB files, Python can save hours of manual effort. Whether you’re an author, a publisher, or simply an e-book enthusiast, Python provides an efficient way to customize your EPUB files.
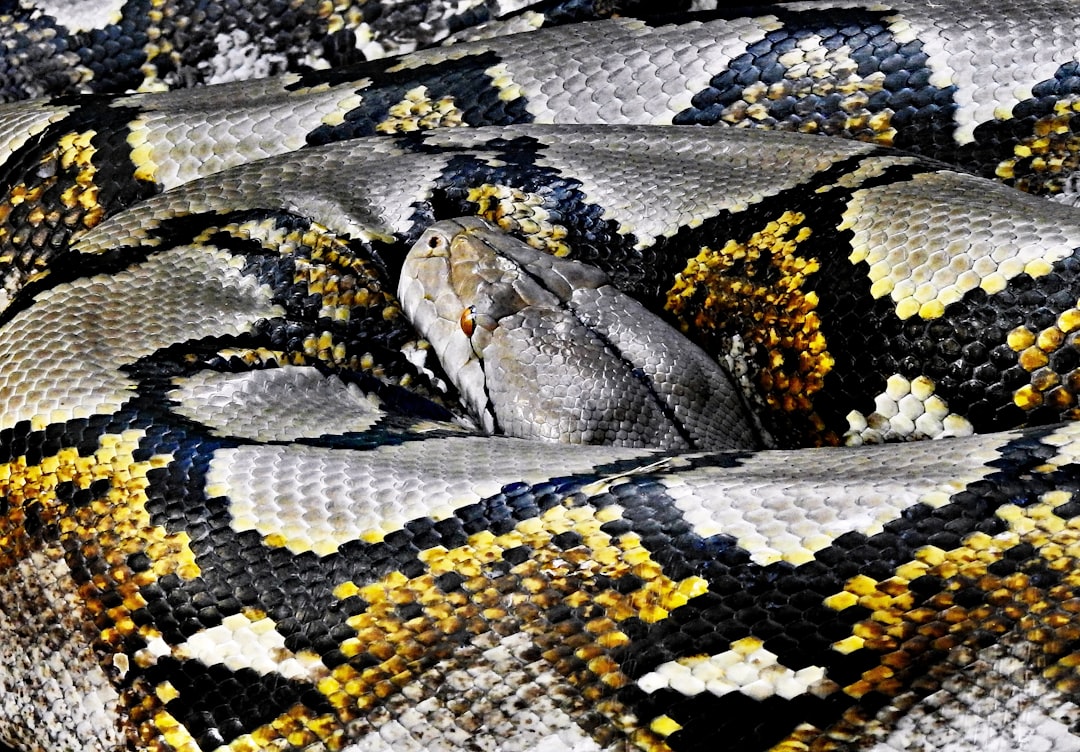
Conclusion
Editing EPUB metadata with Python is a surprisingly simple and effective process. Using tools like EbookLib, you can easily access, modify, and save metadata for your e-books. Whether you’re standardizing formatting across a digital library or adding personal touches to your files, this skill can be incredibly useful in managing your EPUB collection with precision and ease.
So why not give it a try? A few lines of Python code are all it takes to transform how your EPUB files are organized. Happy editing!